Image Processing: Symmetrization
Original Image |
|
Symmetrized |
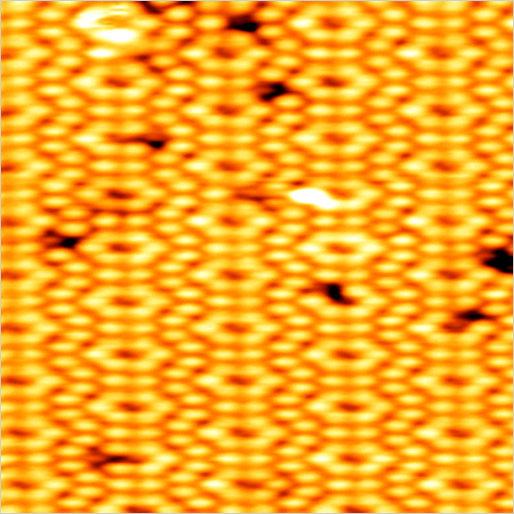 |
➯ |
 |
|
Source Code in Matlab:
This program symmetrizes an image via FFT analysis, following these steps:
- calculate FFT,
- find the maximum-intensity spots,
- fit an ellipse to the FFT spots,
- transform ellipse to circle,
- apply the transformation to image.
1. Read image and calculate FFT
img = imread('image.png'); % Read image
img_fft = fft2(img); % Calculate 2D FFT
img_fft = fftshift(img_fft); % Move zero-frequency components to center
F = abs(img_fft); % Magnitude of FFT
F = log(F+1); % Take log for better visualization
F = (F(:,:,1) + F(:,:,2) + F(:,:,3))/3; % Take the average of RGB channels
F = imfilter(F, fspecial('gaussian')); % Filter the image by Gaussian smoothing
% Display images
figure(1); imshow(img); % Display the original image
figure(2); imagesc(F); % Display FFT
axis image; axis off;
% Zoom in on FFT
xsize = size(img,1);
ysize = size(img,2);
xzoom = xsize*3/8 : xsize*5/8;
yzoom = ysize*3/8 : ysize*5/8;
figure(3); imagesc(F(xzoom, xzoom));
axis image; axis off;
Original Image |
FFT |
FFT Zoomed In |
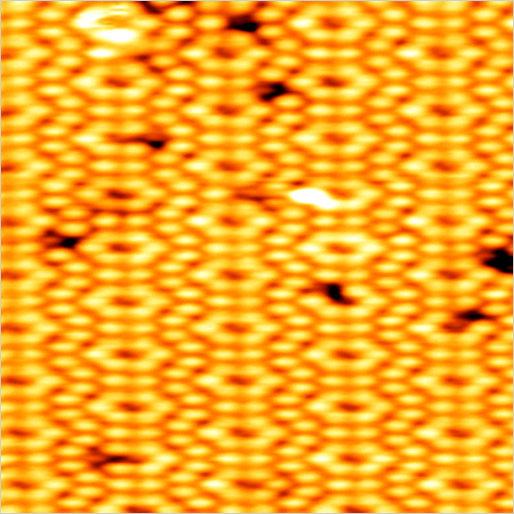 |
 |
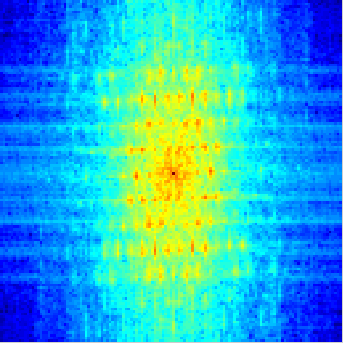 |
Filter out the low- and high-frequency noise
x = (1:xsize) - xsize/2 - 1; % x-coordinates of FFT
y = (1:ysize) - ysize/2 - 1; % y-coordinates of FFT
[X,Y] = meshgrid(x,y); % X and Y coordinate matrices
R = sqrt(X.^2 + Y.^2); % Radius matrix
Rmax = sqrt(xsize^2 + ysize^2) / 2; % Maximum radius
R1 = Rmax/8; % Low freq cut-off
R2 = 10; % High freq cut-off
cutoff = (R > R1) | (R < R2); % Frequency filter
F(cutoff) = min(F(~cutoff)); % Remove low and high frequency components
% Display the filtered FFT
figure(4); imagesc(x,y,F);
axis image; axis off;
xlim([-R1,R1]); ylim([-R1,R1]);
FFT Filtered |
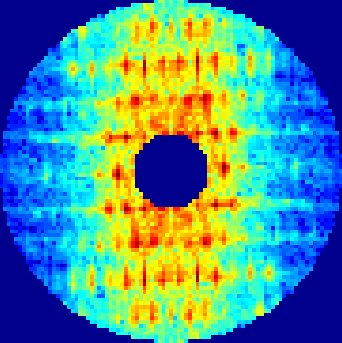 |
2. Find the maximum-intensity FFT spots
spots = struct('x',[],'y',[]); % List of FFT spots
for i = 1:7,
j = find(F==max(F(:))); % Find the maximum of FFT
j = j(1); % Pick the first spot
% Add the selected spot and its mirror image to the list
Xmax = [X(j) -X(j)];
Ymax = [Y(j) -Y(j)];
spots.x = [spots.x Xmax];
spots.y = [spots.y Ymax];
% Display the spots
hold on;
scatter(Xmax,Ymax,100,'w','filled');
scatter(Xmax,Ymax,100,'k');
hold off;
% Remove the selected spots from FFT
rng = abs(X-Xmax(1))<=5 & abs(Y-Ymax(1))<=5;
F(rng) = 0;
rng = abs(X-Xmax(2))<=5 & abs(Y-Ymax(2))<=5;
F(rng) = 0;
end
FFT Spots |
 |